Cloud Actions Getting started videos
Introduction
In this series of videos, you will learn about:
- Cloud Actions concepts (for an overview see Working with Data in the Cloud_Temp_Jan_7_2020.
- Creating a Cloud Action
- Using the Cloud Action Simulator
The sample use case and code used in these videos is provided near the bottom of this page along with some additional enhancements that you can bring to this sample Cloud Action.
Cloud Action concepts
Creating a Cloud Action
Using the Cloud Action Simulator
Reference implementation and code
The sample use case mentioned in the video consists of the following setup, where the Cloud Action :
-
is triggered by Events, reporting devices’ GPS locations (Lat / Lon)
-
retrieves data from a third-party API to get the weather forecast at a device’s location. The third-party service used is https://openweathermap.org/api/one-call-api.
-
publishes data to a third-party API to report device location and predicted weather. The third-party used is https://webhook.site/.
-
reads and writes data to Octave Streams to send the weather forecast to the device’s display:
- the company-level Stream
/company/weather
, is used to store the latest weather forecast sent to any device. The Event'shash
property is used to ensure the Event's uniqueness and to easily find the data stored for a given device when the Cloud Action is executed by using Octave.Event.findHash() - the device-level Stream
/company/devices/<device_name>/:command
is used to send the relevant information to the device's screen
- the company-level Stream
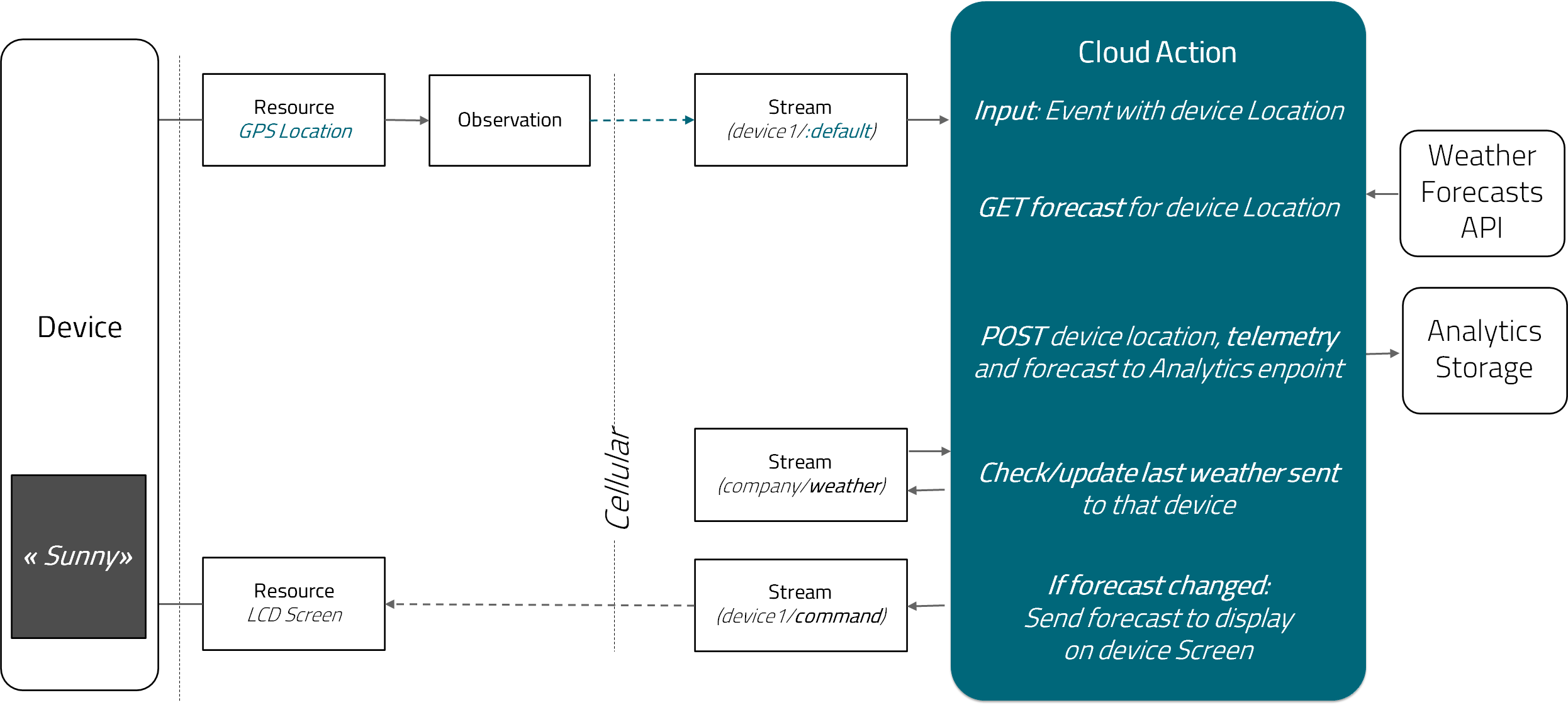
The Cloud Action setup uses Tags:
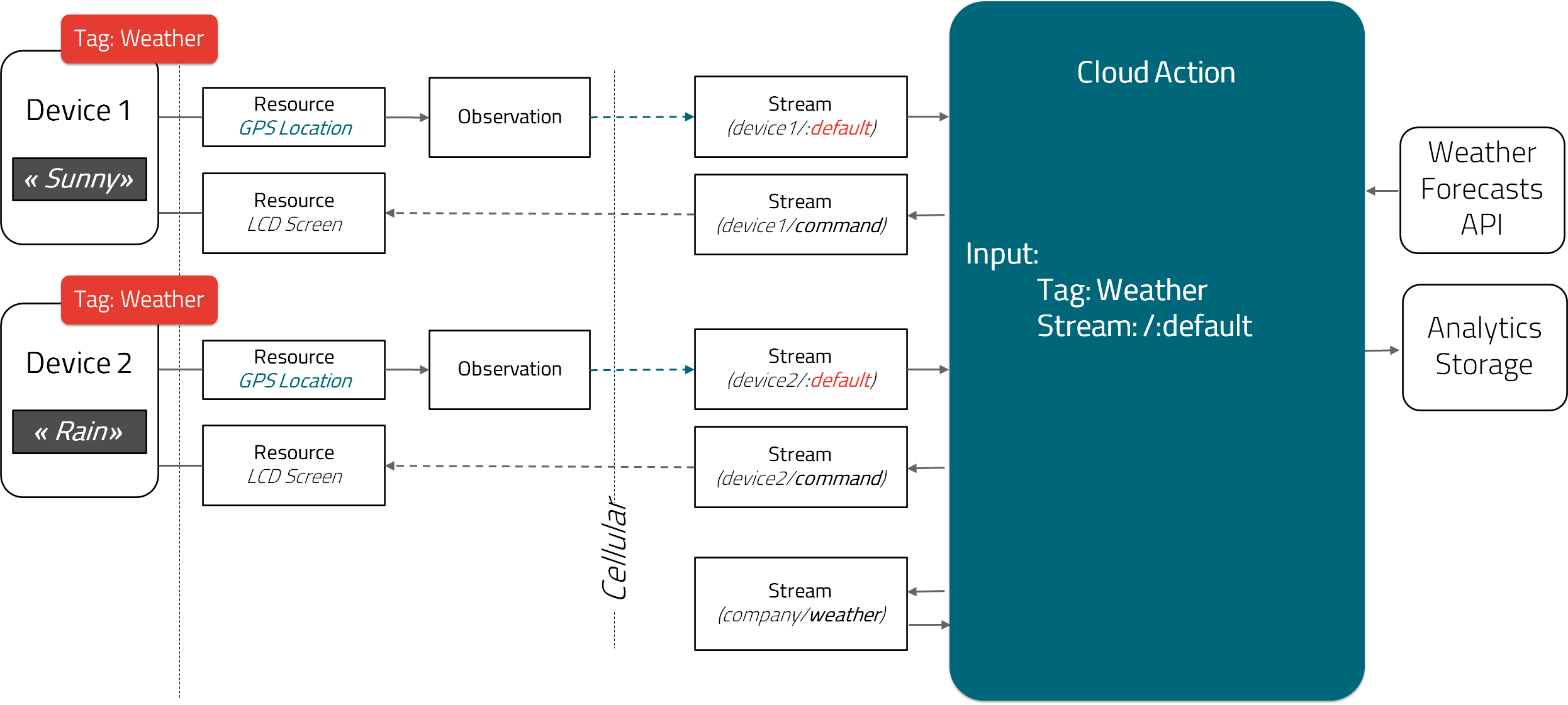
function(event) {
// initialize variables:
var headers={'Content-Type': 'application/json'};
var output = {};
// *** Extract variables from incoming event ***:
var device_lat=event.elems.location.coordinates.lat;
var device_lon=event.elems.location.coordinates.lon;
var deviceId = event.path.split("/")[3];
// *** GET weather for device location ***:
var weather_url = 'https://api.openweathermap.org/data/2.5/onecall?lat='+device_lat+'&lon='+device_lon+'&exclude=hourly,daily,minutely&appid=<you_api_key>';
var weather_response = Octave.Http.get(weather_url,headers);
var weather_forecast = JSON.parse(weather_response.content);
var current_weather = weather_forecast.current.weather[0].description;
console.log("Weather prediction API response is: "+ current_weather);
// *** POST device location and weather to 3rd party cloud *** :
var cloud_payload = {
"Device": deviceId,
"Latitude": device_lat,
"Longitude": device_lon,
"Timestamp": event.generatedDate,
"Forecast weather": current_weather
};
var customer_URL = "https://webhook.site/<your_webhook_endpoint_id>";
var postBody = JSON.stringify(cloud_payload);
var webapi_result = Octave.Http.post(customer_URL, headers, postBody);
// *** Return current weather to the device if required ***
// Search for existing device weather Data in the relevant Weather Stream
var device_weather = Octave.Event.findHash("<stream_id_of_the_weather_cloud_stream>", deviceId).elems.weather;
console.log("Last weather update sent to the device is: "+ device_weather)
// Compare new weather data with existing data and send update to the device if required
if (device_weather!=current_weather){
console.log("Weather predictions need to be updated on the device")
console.log("Returning data to device/:command and weather Streams")
var command_path = "/<company_name>/devices/"+deviceId+"/:command";
var weather_path = "/<company_name>/weather";
output[command_path]= [{"elems": { "/lcd/txt1": "Weather: "+current_weather} }]
output[weather_path]= [{"elems": { "weather": current_weather},"hash":deviceId }]
return output;
}
else {
console.log("Weather predictions do not need to be updated on the device")}
}
Going Further
There are two main areas where this Cloud Action could have been expanded.
The first one consists in handling the Octave.Http
responses to log and/or handle errors. As indicated in the Cloud JavaScript Library documentation, the HTTP response
, status
and headers
are returned. These can be used to handle errors during the execution of the Cloud Action. Writing these responses or errors to another dedicated Stream will allow you to keep track of the Cloud Action's execution.
The other area relates to the use case, which has been kept simple for the sake of clarity. As the weather prediction API provides much more information than a simple forecast description, this information could be sent to the device to allow edge-side logic. For instance, some elements of the weather API response below could be sent to the device, targeting a Virtual Resource. The device could then for example, make use of the predicted temperature or UV index to control HVAC or automated window blinds.
"current": {
"clouds": 2,
"dew_point": 284.58,
"dt": 1600092053,
"feels_like": 305.37,
"humidity": 24,
"pressure": 1017,
"sunrise": 1600062181,
"sunset": 1600107470,
"temp": 308.15,
"uvi": 5.43,
"visibility": 10000,
"weather": [
{
"description": "clear sky",
"icon": "01d",
"id": 800,
"main": "Clear"
}
],
"wind_deg": 240,
"wind_speed": 4.6
},
"lat": 44.49,
"lon": -1.21,
"timezone": "Europe/Paris",
"timezone_offset": 7200
} ```
Updated over 3 years ago